Regular expressions (regex) are powerful tools for pattern matching and text manipulation. Below are some useful regex patterns along with examples to demonstrate their utility.
1. Extracting Strings Enclosed in Single or Double Quotes
Pattern:
/(["'])(?:\\.|[^\\])*?\1/g
Example in PHP:
<?php
$someCode = <<<TXT
protected \$description = 'Command \'description\'';
protected \$signature = "index:reindex 'attr1' \"some text\"";
TXT;
preg_match_all('/(["\'])(?:\\\\.|[^\\\\])*?\1/', $someCode, $matches);
print_r($matches[0]);
Output:
Array
(
[0] => 'Command \'description\''
[1] => "index:reindex 'attr1' \"some text\""
)
2. Extracting Email Addresses from Text
Pattern:
/[A-Za-z0-9._%+-]+@([A-Za-z0-9.-]+\.)+[A-Z|a-z]{2,}/g
Example in PHP:
Example in PHP:
<?php
$text = "Please contact us at [email protected] for more information.";
preg_match_all('/[A-Za-z0-9._%+-]+@([A-Za-z0-9.-]+\.)+[A-Z|a-z]{2,}/', $text, $matches);
print_r($matches[0]);
?>
Output:
Array
(
[0] => [email protected]
)
3. Extracting Sentences Before a Certain Word
Pattern:
/.*?(some words)/iu
Example in PHP:
<?php
$text = "This is a sample text containing some words that we want to match.";
preg_match('/(.*?)(?=some words)/is', $text, $matches);
echo $matches[0];
Output:
This is a sample text containing
4. Extracting <script>
Tags with Their Content
Pattern:
/<script.*?>.*?<\/script.*?>/sig
Example in PHP:
<?php
$html = '<html><head><script type="text/javascript">alert("Hi");</script></head></html>';
preg_match_all('/<script.*?>.*?<\/script.*?>/si', $html, $matches);
print_r($matches[0]);
?>
098
Output:
Array
(
[0] => <script type="text/javascript">alert("Hi");</script>
)
5. Removing All Tag Attributes
Pattern:
/<(\w+)[^>]*>/
Example in PHP:
<?php
$html = '<a href="https://example.com" class="link">Example</a>';
$cleaned = preg_replace('/<(\w+)[^>]*>/', '<$1>', $html);
echo $cleaned;
Example in JavaScript:
const html = '<a href="https://example.com" class="link">Example</a>';
const cleaned = html.replace(/<(\w+)[^>]*>/g, '<$1>');
console.log(cleaned);
Output:
<a>Example</a>
Conclusion
These regex patterns provide practical solutions for common tasks such as extracting quoted strings, email addresses, and script tags, as well as cleaning up HTML. Practice these patterns to strengthen your understanding of regex and explore its powerful applications in text processing.
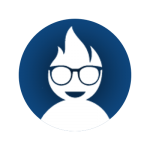
Post a comment